SymPy¶
SymPy (http://www.sympy.org/) is a Python module for symbolic mathematics. It’s similiar to commercial computer algebra systems like Maple or Mathematica.
Usage¶
Python Module¶
SymPy can be installed, imported and used like any other regular Python module.
Output can be done as nicely formatted LaTeX. This is a great way to get more complicated formulae into your manuscript insted of hassling with nested LaTeX commands. Right click on the outputted formula in any ipython notebook or the above mentioned web services to extract the corresponding LaTeX commands.
Basic Examples¶
%matplotlib inline
from sympy import *
Value error parsing header in AFM: b'UnderlinePosition' b'-133rUnderlineThickness 20rVersion 7.000'
init_printing() activates nicely formatted LaTeX output within an ipython notebook.
init_printing()
Symbols¶
Mathematical variables have to be defined as symbols before usage
x = Symbol('x')
type(x)
sympy.core.symbol.Symbol
(pi + x)**2
A short form of Symbol is S. Both can be used to define serveral symbols at once.
x,y,z = S('x,y,z')
x**2+y**2+z**2
Fractions¶
Fractions of nubers must be defined with Rational. Otherwise the numbers will be evaluated as a Python expression resulting in a regular Python floating point number.
4/7 # regular python division
r1 = Rational(4,7) # sympy rational
r1
r2 = x/y # since x and y are already sympy symbols no explicit Rational definition is required
r2
r1*r2+1
Complex Numbers¶
The imaginary unit is noted as I in sympy.
1+I
I**2
simplify((1+I)**2)
Numeric evaluation¶
r1.evalf(100)
pi.evalf(100)
Substitution¶
f1 = x**2+x**3
subs can be used to evaluate a function at a specific point
f1.subs(x, 2)
or to substitute expressions
a=S('a')
f1.subs(x, a)
f2 = f1.subs(x, sqrt(a))
f2
f2.subs(a, 1)
Plotting¶
from sympy.plotting import plot
f1
plot(f1)
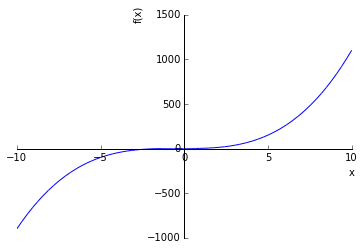
<sympy.plotting.plot.Plot at 0x7f1b3125a5f8>
plot(f1, (x,-5,5))
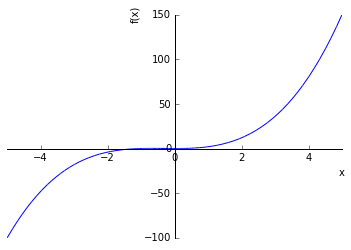
<sympy.plotting.plot.Plot at 0x7f1b11812e48>
f2
plot( f2)
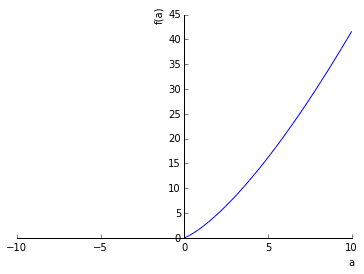
<sympy.plotting.plot.Plot at 0x7f1b117e2898>
Simplification¶
x,y=S('x,y')
eq = (x**3 + x**2 - x - 1)/(x**2 + 2*x + 1)
eq
simplify(eq)
simplify(sin(x)**2 + cos(x)**2)
Solving equations¶
x = S('x')
eq = x**2 - 1
eq
plot(eq, (x, -2, +2))
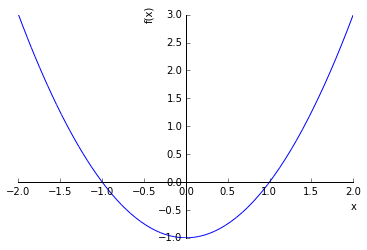
<sympy.plotting.plot.Plot at 0x7f1b117665f8>
solve(eq, x)
eq = 2*x*y + y**2/2
solve(eq, y)
Matrices¶
unity matrix
eye(10)
m11, m12, m21, m22 = symbols("m11, m12, m21, m22")
b1, b2 = symbols("b1, b2")
A = Matrix([[m11, m12],[m21, m22]])
A
b = Matrix([[b1], [b2]])
b
A**2
A * b
A.det()
A.inv()
Example: Calculate determinant of matrices in a loop¶
from IPython.display import display
#b01a01
A = Matrix( [[2,2,-5],[5,4,1],[4,14,3]]) # assign matrix to variable A
B = Matrix( [[1,2,3],[0,4,5],[0,0,6]]) # assign matrix to variable B
C = Matrix( [[1,2,-1,2],[-3,5,-5,-2],[5,4,3,-4], [-4,-3,5,5]]) # assign matrix to variable C
# show the result for each matrix
for M in (A, B, C):
print( 'The determinant of matrix')
display(M)
print(' is ')
display(M.det())
The determinant of matrix
is
The determinant of matrix
is
The determinant of matrix
is
Example: Calculate Eigenvalues and Eigenvectors¶
a,b,c,d = S('a,b,c,d')
A = Matrix( [[a,b], [c,d]])
A
A.eigenvals()
A.eigenvects()
B = A.subs(((a,1),(d,1)))
B
B.eigenvals()
B.eigenvects()